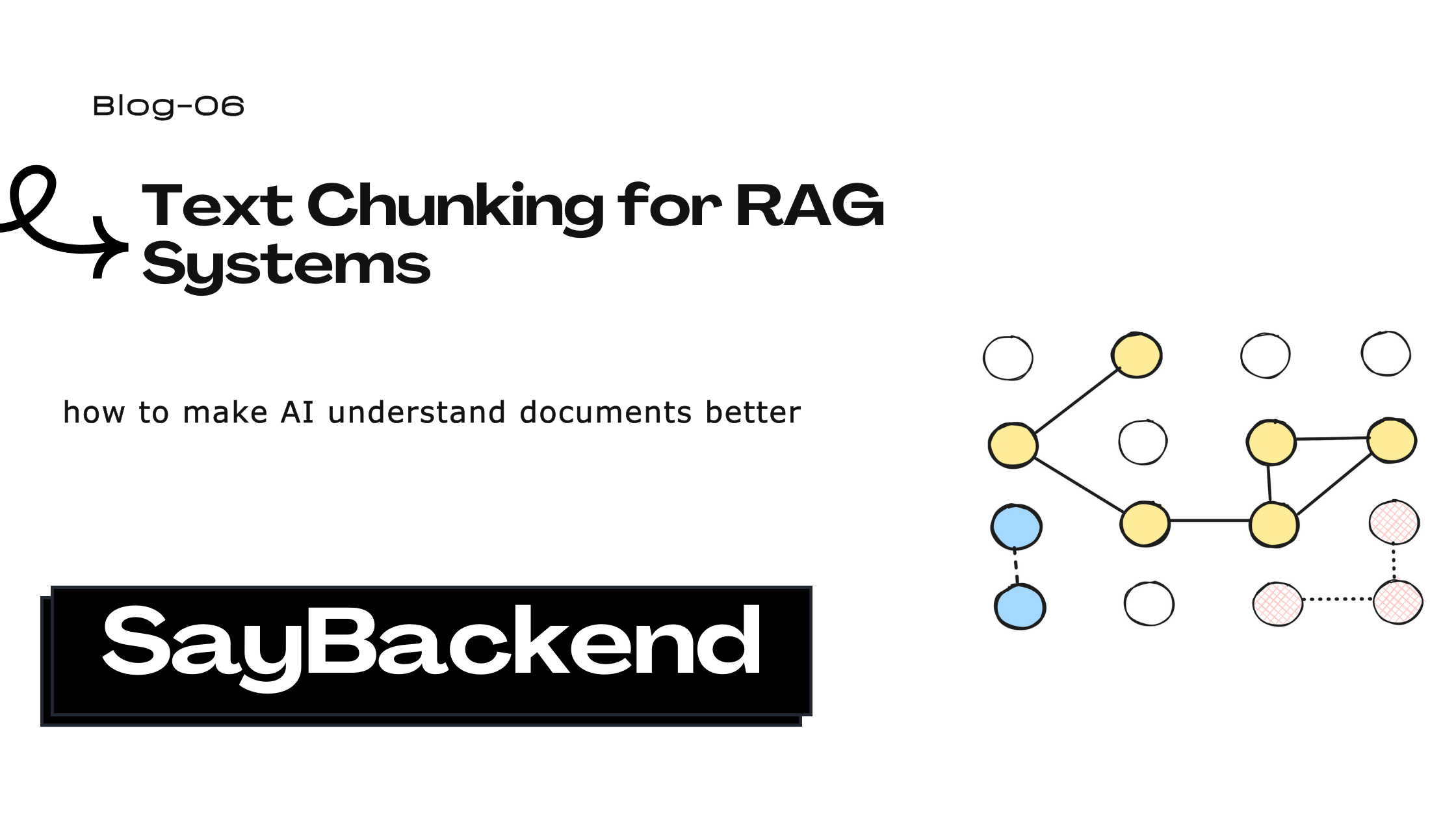
Text Chunking for RAG Systems: How to Make AI Understand Documents Better
Think of reading a book through a keyhole. Youād catch bits and pieces, but never the full story. Thatās exactly what happens when AI breaks text into chunks the wrong way. In Retrieval-Augmented Generation (RAG) systems, how you divide documents can make or break the quality of your results. Letās look at some practical ways to help AI get the clearest, most useful view of your data.
My Experience with Chunking
Not long ago, I had to build a RAG system from scratch. I knew just enough about embeddings to realize I needed better chunking. So, I went deepāreading research papers, watching YouTube breakdowns, and learning from experts. The more I learned, the more I realized how huge of a difference proper chunking makes.
Hereās what I found.
Why Chunking Matters
A RAG system works in two main steps:
- Learning Phase ā Breaking a document into structured, meaningful pieces that can be stored.
- Answering Phase ā Pulling the right chunks to generate accurate, relevant answers.
When chunking goes wrong, you get:
- Choppy, disconnected ideas (āI love lanā¦guage processing?ā)
- Confusing answers that mix unrelated info
- Slower responses due to extra, unnecessary data
But when done right:
- Ideas stay intact so AI gets the full context
- Search is faster and more precise
- Answers actually make sense
Chunking isnāt just a small detailāitās a game-changer for any RAG system. Up next, letās dig into how to do it right.
5 Ways to Split TextāFrom Simple to Sophisticated
You donāt need to use langchain since these are very basic strategies and can code them yourself.
1. Cookie-Cutter Splitting (Character Limits)
Best for quick prototypes. Chops text every X characters like slicing bread. Fast but messy.
from langchain.text_splitter import CharacterTextSplitter
article_content = "Effective text segmentation acts as a cognitive aid for language models."
chopper = CharacterTextSplitter(chunk_size=25, chunk_overlap=8)
document_slices = chopper.split_text(article_content)
print("Cookie-cutter slices:", document_slices)
Output:
['Effective text segmen', 'egmenation acts as a', 's a cognitive aid for', 'r language models.']
Watch out for: Split terms like āsegmen|tationā losing meaning.
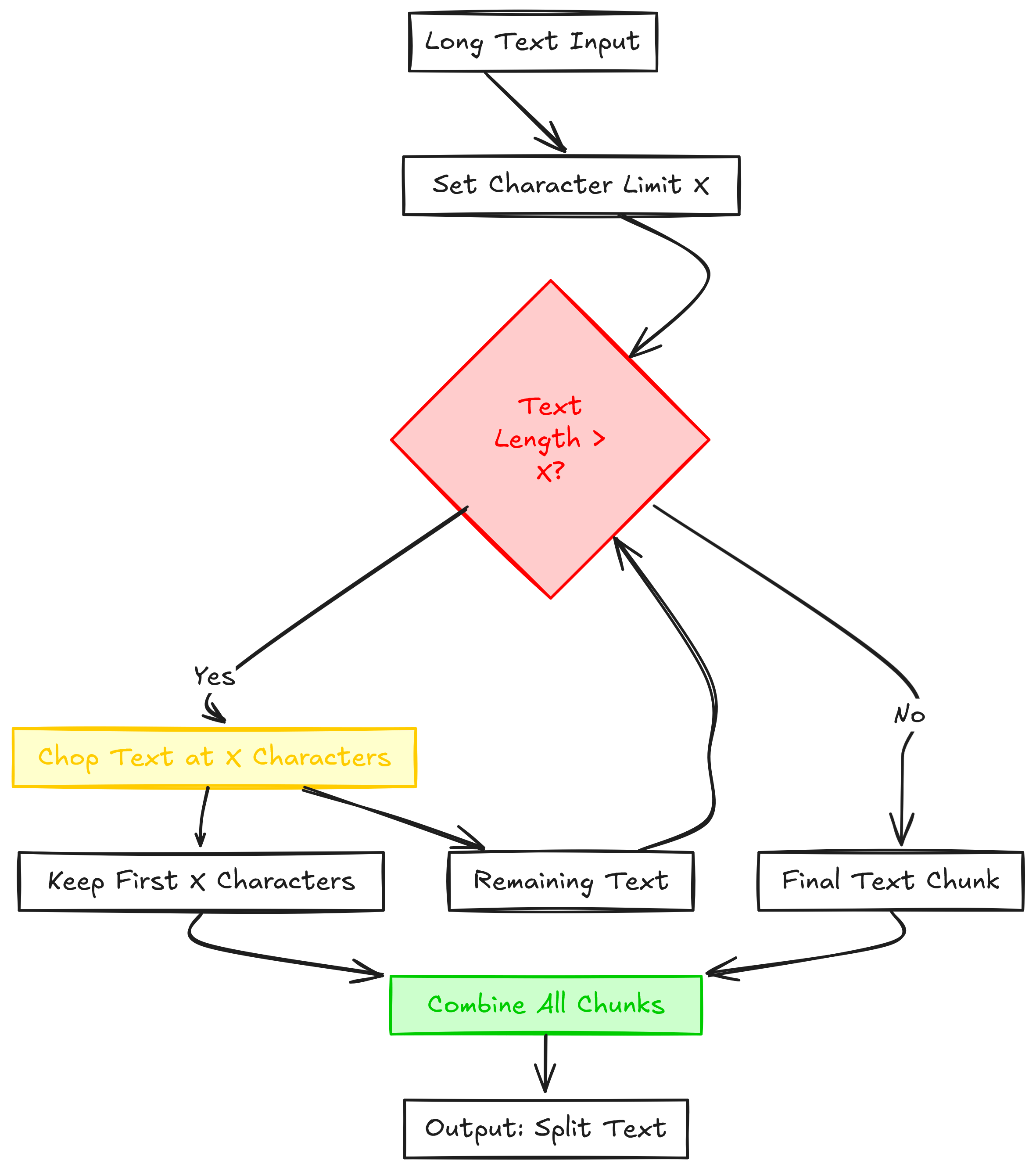
2. Natural Breaks Splitting (Paragraphs & Sentences)
Great for articles & reports. Respects existing structure like paragraphs and punctuation.
from langchain.text_splitter import RecursiveCharacterTextSplitter
research_paper = """
Modern NLP requires careful data preparation.
Transformer models like BERT need clean input.
Proper chunking improves model performance significantly.
"""
smart_splitter = RecursiveCharacterTextSplitter(chunk_size=150, chunk_overlap=25)
logical_chunks = smart_splitter.split_text(research_paper)
print("Natural-break chunks:", logical_chunks)
Output:
['Modern NLP requires careful data preparation.',
'Transformer models like BERT need clean input.',
'Proper chunking improves model performance significantly.']
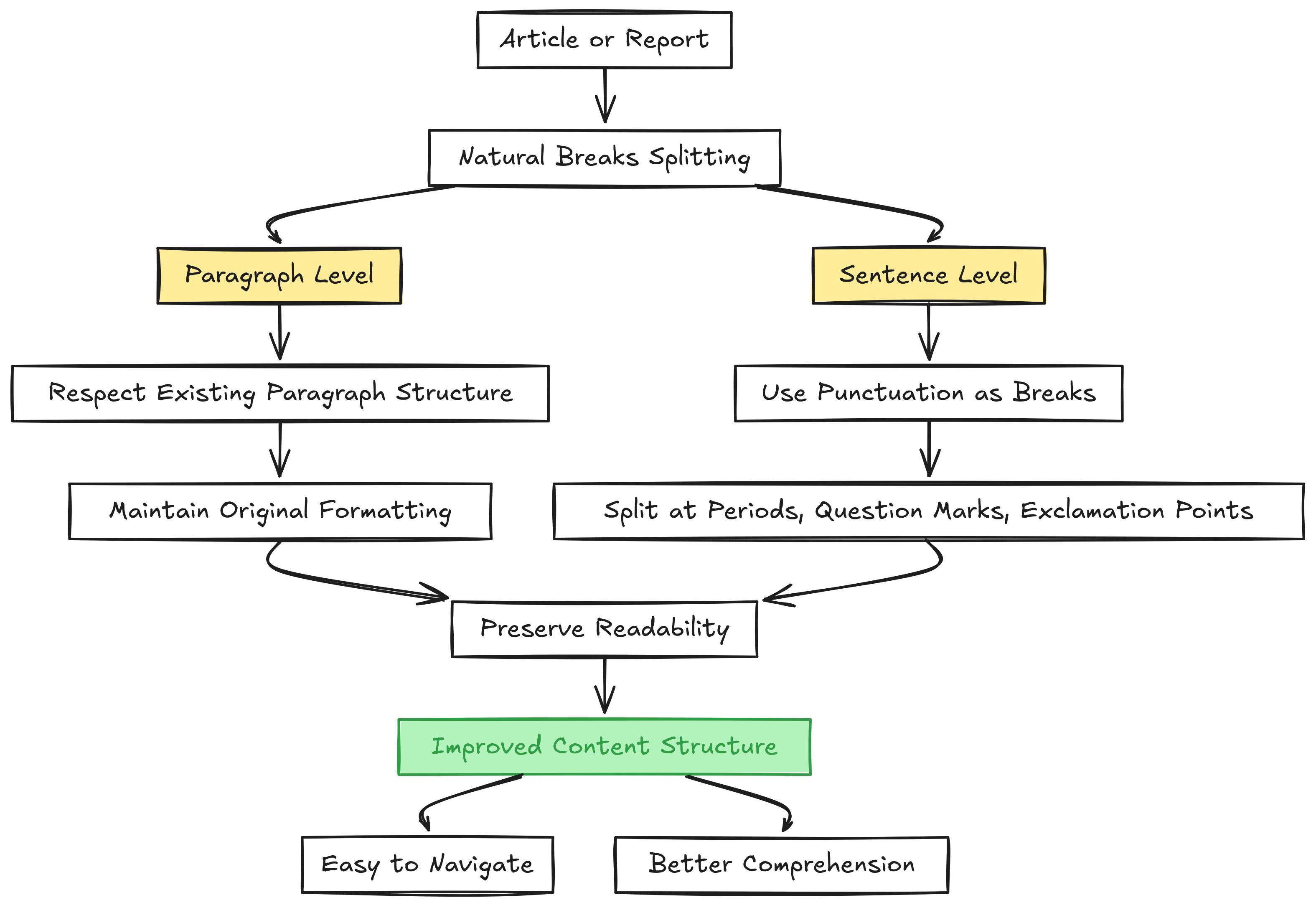
3. Structure-Aware Splitting (For Technical Docs)
Perfect for code, markdown, or HTML. Uses document formatting as chunk boundaries.
from langchain.text_splitter import MarkdownTextSplitter
technical_guide = """
## API Documentation
### Authentication
- Use OAuth2.0 tokens
- Token expires every 3600 seconds
### Rate Limits
- 100 requests/minute
- Exponential backoff recommended
"""
doc_splitter = MarkdownTextSplitter(chunk_size=200)
section_chunks = doc_splitter.split_text(technical_guide)
print("Structured chunks:", section_chunks)
Output:
['## API Documentation\n\n### Authentication',
'- Use OAuth2.0 tokens\n- Token expires every 3600 seconds',
'### Rate Limits\n- 100 requests/minute\n- Exponential backoff recommended']
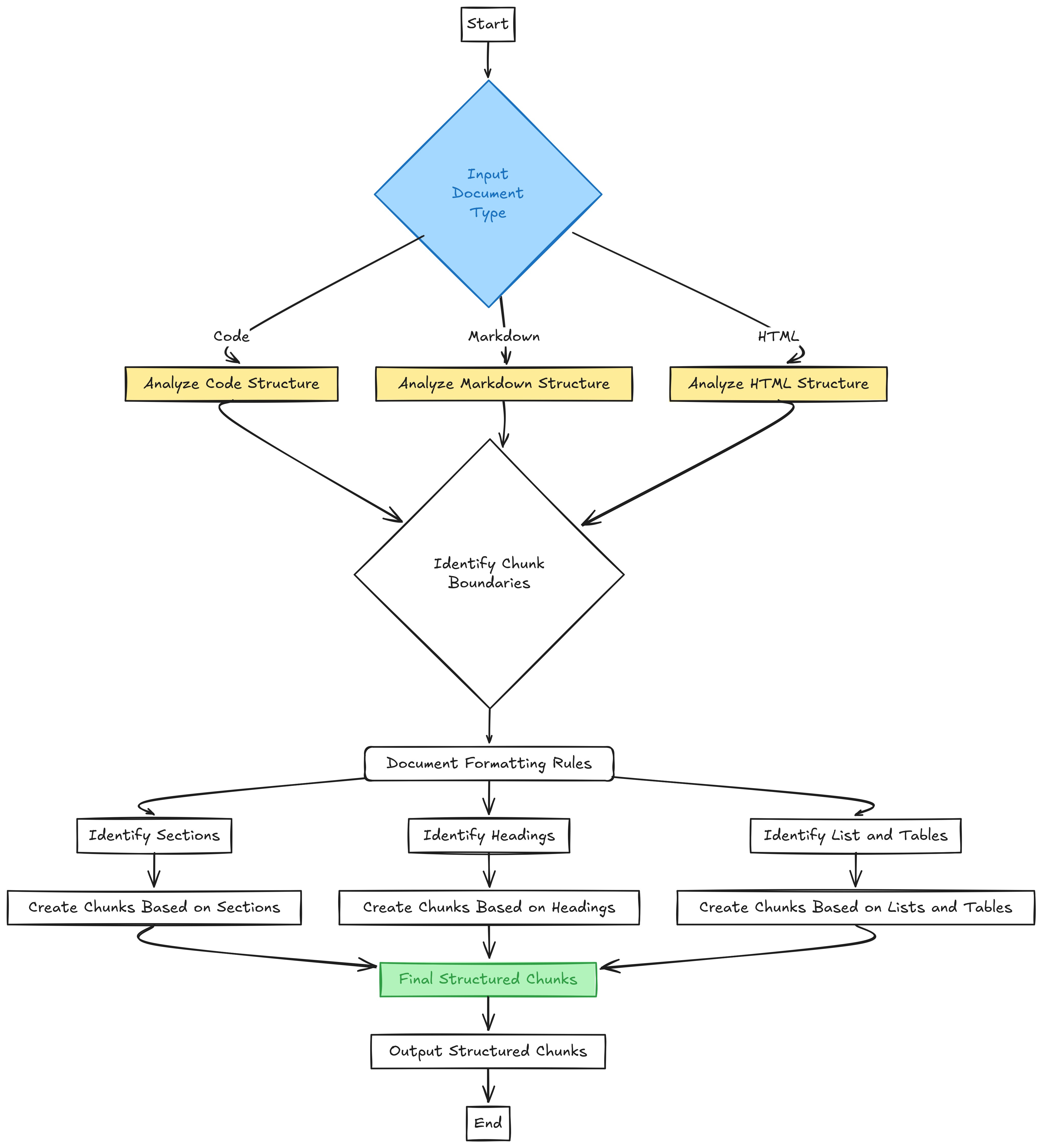
4. Meaning-Based Chunking (Semantic Grouping)
Ideal for complex concepts. Clusters text by ideas rather than fixed rules.
from langchain_experimental.text_splitter import SemanticChunker
from langchain_openai.embeddings import OpenAIEmbeddings
philosophy_text = """
Knowledge representation challenges AI systems.
Vector databases enable semantic similarity searches.
Together they form modern information retrieval systems.
"""
meaning_splitter = SemanticChunker(OpenAIEmbeddings())
idea_clusters = meaning_splitter.split_text(philosophy_text)
print("Conceptual groups:", idea_clusters)
Output:
['Knowledge representation challenges AI systems.',
'Vector databases enable semantic similarity searches.',
'Together they form modern information retrieval systems.']
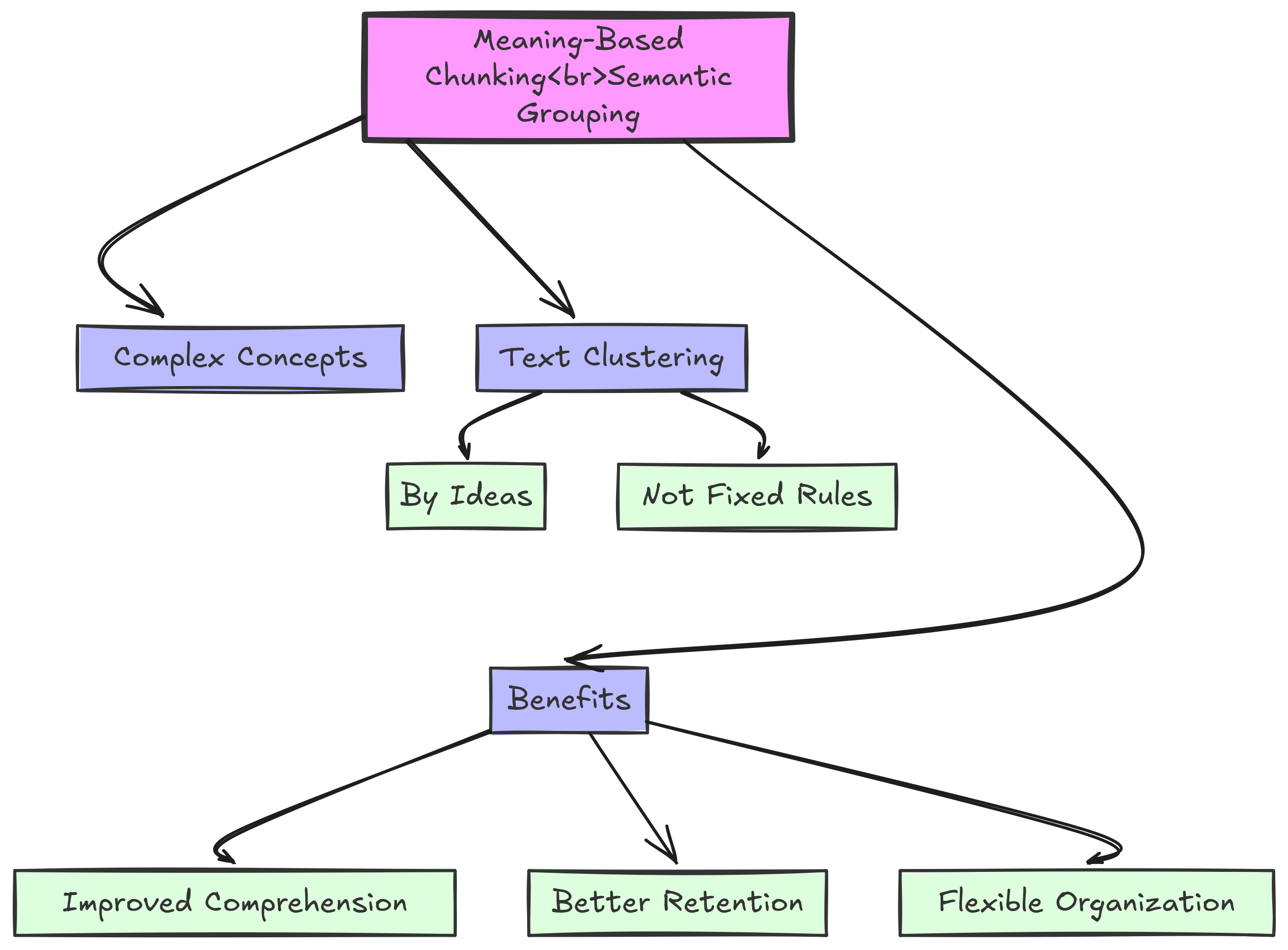
5. Adaptive Chunking (AI-Powered Grouping)
For cutting-edge applications. Uses LLMs to dynamically organize content.
This is a hypothetical example. And get the content blocks from the semantic chunker above.
AdaptiveGrouper is a hypothetical advanced module. You will need to implement this in your own code. Use LLMs to generate the titles, summary, and group type for each content block. The aim will be that these chunks work well separately and together. Like the chunks from the semantic chunker and the grouped summarized ones in the adaptive grouper.
from custom_context_engine import AdaptiveGrouper # Hypothetical advanced module
content_blocks = [
"Neural networks require quality training data.",
"Embedding models convert text to numerical vectors.",
"These components power modern semantic search systems."
]
context_organizer = AdaptiveGrouper()
for block in content_blocks:
context_organizer.analyze_content(block)
smart_groups = context_organizer.generate_clusters()
print("Adaptive clusters:", smart_groups)
Output:
[Document(content='Neural networks require quality training data. These components power modern semantic search systems.', metadata={'group_type': 'technical_concepts'}),
Document(content='Embedding models convert text to numerical vectors.', metadata={'group_type': 'implementation_details'})]
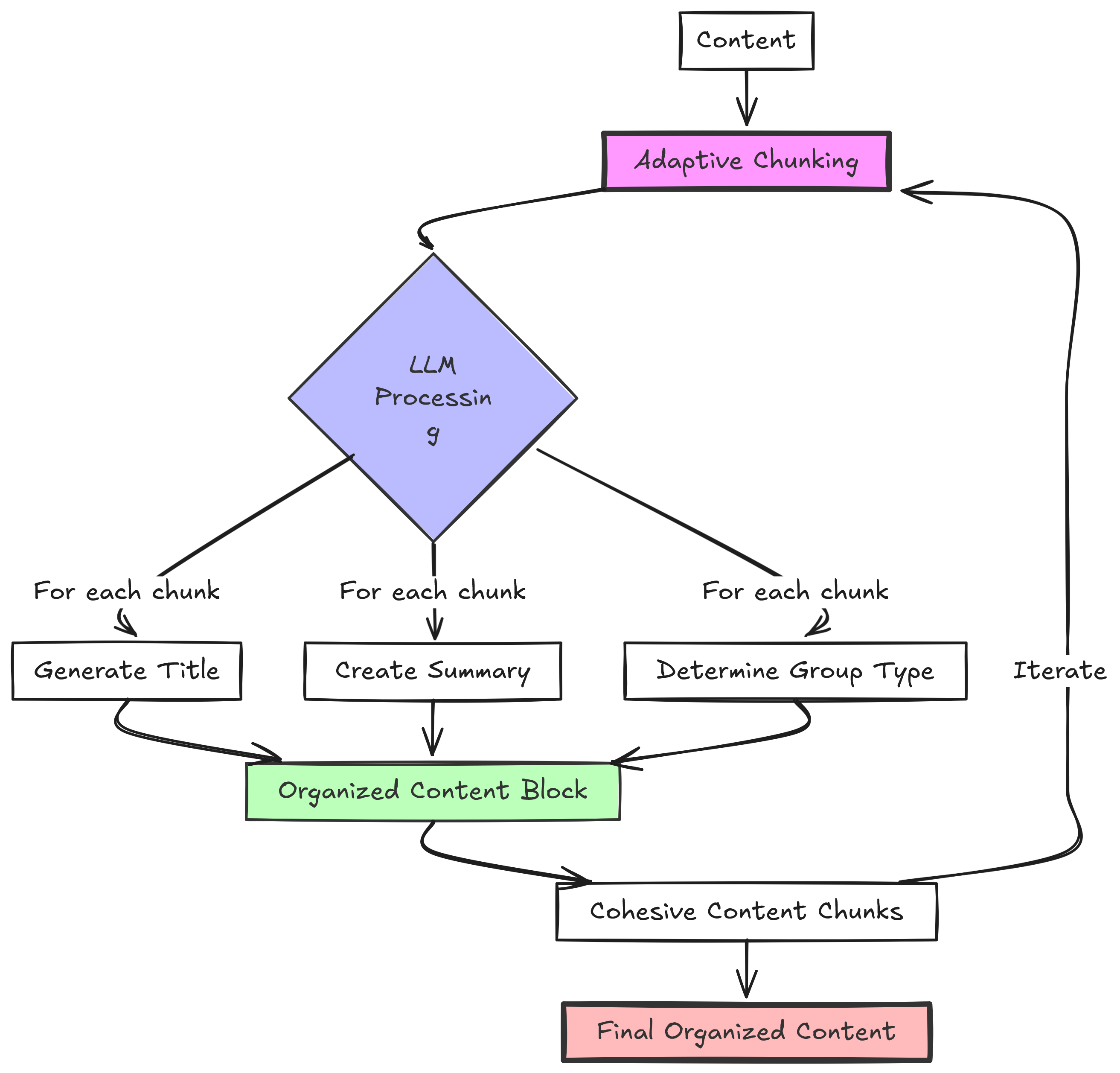
Choosing Your Chunking Strategy
Method | Best For | Complexity | Context Preservation | Cost |
---|---|---|---|---|
Cookie-Cutter | Quick prototypes | Low | ā | Low |
Natural Breaks | Articles & reports | Medium | āāāā | Medium |
Structure-Aware | Technical documentation | Medium | āāāāā | Medium |
Meaning-Based | Research papers | High | āāāāā | High |
Adaptive | Enterprise knowledge systems | Very High | āāāāā | Very High (Use prompt caching to reduce cost) |
Pro Tip: Start simple and scale up. Most applications do well with natural breaks splitting, while technical docs benefit from structure-aware approaches. Save adaptive chunking for mission-critical systems.
Remember: The best chunking strategy mirrors how humans naturally process informationākeeping related ideas together while maintaining manageable piece sizes. Test different approaches and monitor how they affect your AIās performance!
Nowadays, the LLMs are very cheap, very fast, and have a very big context window, so much so that you can almost put whole document in it at once. So you donāt need to create small small chunks, try pairing the semantic chunking with like 10,000 tokens chunks with the AdaptiveGrouper.
It also depends on your embedding model, and nowadays, even the models that you can run locally like BGE-m3, nomic-embed-text, are more than capable of handling the whole document at once. Or use the Gemini free embedding API.